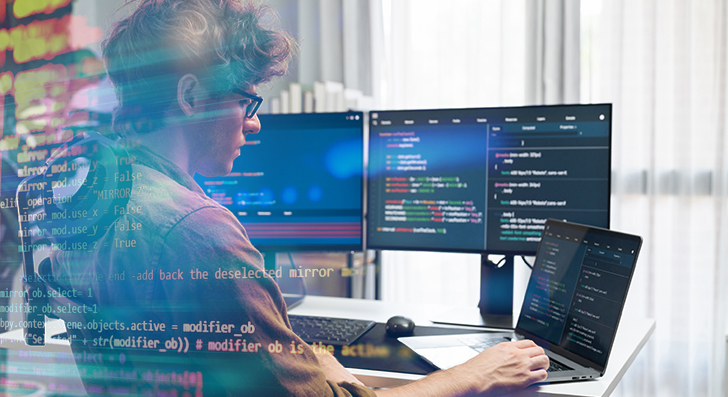
Scalability implies your application can deal with growth—extra people, far more information, and much more traffic—without breaking. For a developer, creating with scalability in mind will save time and stress afterwards. Right here’s a transparent and functional manual to help you start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your prepare from the start. Several purposes fall short every time they expand fast due to the fact the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Avoid monolithic codebases in which all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into smaller, independent areas. Each individual module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day a single. Will it want to manage one million users or simply a hundred? Select the right kind—relational or NoSQL—depending on how your data will improve. Plan for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A further essential level is in order to avoid hardcoding assumptions. Don’t create code that only operates beneath latest disorders. Consider what would come about In the event your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure patterns that assistance scaling, like message queues or function-pushed systems. These support your application take care of far more requests with no finding overloaded.
After you Establish with scalability in mind, you are not just preparing for success—you are reducing future head aches. A effectively-prepared procedure is less complicated to take care of, adapt, and grow. It’s improved to prepare early than to rebuild later.
Use the proper Database
Choosing the ideal databases can be a crucial A part of building scalable purposes. Not all databases are built a similar, and utilizing the Improper you can sluggish you down and even cause failures as your application grows.
Commence by comprehending your details. Can it be remarkably structured, like rows within a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great suit. These are solid with relationships, transactions, and regularity. In addition they assistance scaling procedures like read through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your details is more adaptable—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing large volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more effortlessly.
Also, look at your study and publish styles. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you handling a major create load? Investigate databases that can manage significant create throughput, as well as event-dependent details storage techniques like Apache Kafka (for momentary information streams).
It’s also sensible to Imagine ahead. You may not need to have Highly developed scaling features now, but selecting a database that supports them signifies you gained’t need to switch later on.
Use indexing to speed up queries. Keep away from avoidable joins. Normalize or denormalize your data based on your accessibility patterns. And often keep an eye on database general performance when you mature.
In short, the right databases depends on your application’s composition, velocity desires, And just how you assume it to mature. Choose time to select correctly—it’ll help save a great deal of problems later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny hold off adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by composing clear, straightforward code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t choose the most complex Option if an easy one is effective. Maintain your functions brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too very long to run or takes advantage of excessive memory.
Following, look at your databases queries. These frequently gradual issues down much more than the code itself. Be certain Each and every question only asks for the data you really need. Stay clear of Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout big tables.
When you recognize the exact same information staying asked for repeatedly, use caching. Keep the effects temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application much more effective.
Remember to take a look at with significant datasets. Code and queries that work good with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These steps help your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with far more end users and a lot more website traffic. If all the things goes as a result of a person server, it will eventually rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these applications enable keep the application quickly, steady, and scalable.
Load balancing spreads incoming visitors across multiple servers. In lieu of a person server performing all the do the job, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-primarily based options from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused promptly. When end users request the exact same data once more—like an item webpage or perhaps a profile—you don’t really need to fetch it through the database every time. You may serve it within the cache.
There are 2 common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static documents near the consumer.
Caching cuts down database load, enhances speed, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does improve.
In brief, load balancing and caching are uncomplicated but potent equipment. Alongside one another, they help your app cope with much more end users, continue to be quick, and Get well from complications. If you plan to expand, you require the two.
Use Cloud and Container Applications
To build scalable programs, you may need applications that permit your app expand simply. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess potential ability. When targeted traffic boosts, you could increase extra resources with just a few clicks or automatically using automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to developing your app instead of managing infrastructure.
Containers are A further critical Resource. A container deals your app and everything it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app in between environments, from a laptop computer for the cloud, with out surprises. Docker is the most popular Resource for this.
Whenever your app takes advantage of a number of containers, resources like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate aspects of your app into services. You may update or scale elements independently, which is perfect for performance and dependability.
In brief, using cloud and container instruments indicates you could scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you need your application to expand without the need of limitations, start out using these equipment early. They save time, minimize hazard, and assist you to keep focused on constructing, not correcting.
Keep track of Anything
If you don’t check your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location issues early, and make far better selections as your application grows. It’s a vital A part of constructing scalable units.
Begin by tracking standard metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control how much time it's going to take for buyers to load internet pages, how frequently faults take place, and the place they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified immediately. This allows you take care of difficulties rapidly, typically just before customers even observe.
Monitoring is also practical after you make improvements. In case you deploy a fresh function and find out a spike in problems or slowdowns, you'll be able to roll it back in advance of it brings about actual damage.
As your application grows, site visitors and data maximize. With no monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right tools in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not almost recognizing failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant firms. Even small apps have to have a powerful Basis. By creating thoroughly, optimizing wisely, and utilizing the correct instruments, you are able to Create applications that expand effortlessly with out breaking under pressure. Get started little, Consider big, website and Construct clever.